How to Configure Odoo 15 in Visual Studio Code on Ubuntu 20.04: A Step-by-Step Guide
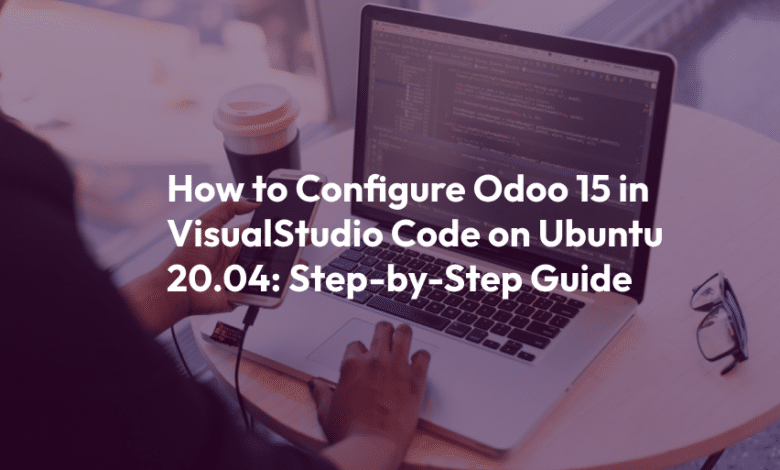
Introduction
Odoo is a popular open-source business management software that combines a wide range of business applications, including CRM, e-commerce, accounting, inventory management, and more. To customize and develop Odoo modules, a robust development environment is crucial. Visual Studio Code (VS Code) is an excellent choice for this purpose, providing a feature-rich integrated development environment (IDE). In this step-by-step guide, we will walk you through the process of configuring Odoo 15 in Visual Studio Code on Ubuntu 20.04, enabling you to set up a productive development environment for Odoo.
Prerequisites
Before diving into the installation and configuration process, you need to make sure you have the following prerequisites in place:
1. Ubuntu 20.04: You should have Ubuntu 20.04 installed and up-to-date.
2. Visual Studio Code: Install VS Code on your Ubuntu system. You can download it from the official website or install it via the Software Center.
3. Python 3.7+: Odoo 15 requires Python 3.7 or higher. You can check your Python version by running `python3 –version`. If it’s not installed, you can install it using `sudo apt install python3`.
4. PostgreSQL Database: Odoo uses PostgreSQL as its database backend. You can install it with the following command:
sudo apt install postgresql
5. Git: You need Git to clone Odoo repositories and extensions. If you don’t have Git installed, you can do so with the following command:
sudo apt install git
Now that you have all the prerequisites ready, let’s configure Odoo 15 in Visual Studio Code.
Step 1: Install Required Dependencies
Odoo relies on several Python libraries and system dependencies. To ensure a smooth installation, you need to install these dependencies using the following command:
sudo apt-get install python3-dev libxml2-dev libxslt1-dev zlib1g-dev libsasl2-dev libldap2-dev build-essential libssl-dev libffi-dev libmysqlclient-dev libjpeg-dev libopenjp2-7 libtiff5 libjpeg8 libjpeg62-turbo
Step 2: Set Up a Virtual Environment
It’s best practice to create a virtual environment for your Odoo project. A virtual environment helps you manage dependencies and isolate your project from the system’s Python environment. To create a virtual environment, follow these steps:
1. Install the virtual environment tool:
pip3 install virtualenv
2. Create a directory for your Odoo project and navigate to it:
mkdir odoo_project
cd odoo_project
3. Create a virtual environment within the project directory:
virtualenv venv
4. Activate the virtual environment:
source venv/bin/activate
Step 3: Clone Odoo Repository
Now, let’s clone the Odoo repository from GitHub. Odoo has a public repository with all the source code you need for your development work. You can clone the repository with the following command:
git clone https://www.github.com/odoo/odoo --depth 1 --branch 15.0 --single-branch .
Step 4: Install Python Dependencies
While your virtual environment is active, install the necessary Python packages for Odoo using pip:
pip install -r requirements.txt
Step 5: Configure Odoo Settings
In your Odoo project directory, you need to create a configuration file that specifies your database connection settings, add-ons, and other configurations. You can start by copying the default configuration file and making the necessary adjustments:
cp odoo.conf odoo.conf
Edit the `odoo.conf` file using your favorite text editor, and make the following changes:
- Set the `admin_passwd` to a secure password.
- Configure the database connection settings, such as `db_host`, `db_port`, `db_user`, and `db_password`.
- Modify other settings as needed for your project.
Step 6: Install PostgreSQL Dependencies
Odoo requires PostgreSQL as its database backend. You should create a PostgreSQL user and database for your Odoo project. Here’s how you can do it:
1. Access the PostgreSQL interactive terminal as the `postgres` user:
sudo -u postgres psql
2. Create a new PostgreSQL user (replace `odoo_user` and `password` with your preferred values):
CREATE USER odoo_user WITH PASSWORD 'password' CREATEDB;
3. Create a new PostgreSQL database, making the user you just created the owner (replace `odoo_db` with your preferred database name):
CREATE DATABASE odoo_db OWNER odoo_user;
4. Grant necessary privileges to the user:
ALTER ROLE odoo_user SUPERUSER;
5. Exit the PostgreSQL interactive terminal:
\q
Step 7: Install Node.js and LessCSS
Odoo uses LessCSS for styling. To compile LessCSS, you need Node.js and npm (Node Package Manager). You can install them as follows:
1. Install Node.js and npm:
sudo apt install nodejs npm
2. Install LessCSS:
npm install -g less less-plugin-clean-css
Step 8: Launch Odoo Server
With all the dependencies in place, you can now start the Odoo server. Make sure your virtual environment is activated, and you are in your project directory. Run the following command:
./odoo-bin -c odoo.conf
You should see the Odoo server starting and listening on a port (usually 8069).
Step 9: Access Odoo via Web Browser
Open your web browser and access Odoo by navigating to `http://localhost:8069`. You should see the Odoo login page. Use the `admin_passwd` and database credentials you set in the `odoo.conf` file to log in.
Step 10: Configure Visual Studio Code
To make your development process smoother, configure Visual Studio Code for Odoo development:
1. Install the Python extension for VS Code to enhance Python development capabilities.
2. Install the Docker extension if you plan to work with Odoo Docker containers.
3. Install the Odoo snippets extension for convenient code completion and snippets for Odoo development.
4. Set up version control in VS Code if you want to track your changes with Git. You can use the GitLens extension for a more powerful Git experience.
5. Configure your workspace settings and editor preferences as per your requirements.
Step 11: Creating Your First Odoo Module
Now that you have Odoo and Visual Studio Code set up, it’s time to create your first Odoo module. Follow these steps to create a simple module that adds a custom field to the Odoo Contacts model:
1. In your project directory, create a new directory for your module. Let’s name it `custom_module`.
mkdir custom_module
2. Inside the `custom_module` directory, create a Python file for your module. You can use the following command to create a Python file with the module’s name, such as `contacts_custom.py`.
touch contacts_custom.py
3. Edit the `contacts_custom.py` file in your text editor and create a basic Odoo module with a custom field:
from odoo import models, fields
class Contact(models.Model):
_inherit = 'res.partner'
custom_field = fields.Char(string="Custom Field")
4. Create an Odoo manifest file (`.xml`) for your module. In your `custom_module` directory, create a new file called `__manifest__.py` and add the following content:
{
'name': 'Custom Contacts Module',
'summary': 'Adds a custom field to the Contacts model',
'description': 'This module adds a custom field to the Contacts model in Odoo.',
'author': 'Your Name',
'website': 'https://www.example.com',
'category': 'Uncategorized',
'version': '1.0',
'depends': ['base'],
'data': [
'views/contacts_custom.xml',
],
}
5. Create a view file for your module. In the `custom_module` directory, create a new directory called `views` and inside it, create a file named `contacts_custom.xml`. Add the following content:
<?xml version="1.0" encoding="UTF-8"?>
<odoo>
<record id="view_res_partner_custom_form" model="ir.ui.view">
<field name="name">res.partner.custom.form</field>
<field name="model">res.partner</field>
<field name="inherit_id" ref="base.view_partner_form"/>
<field name="arch" type="xml">
<field name="function" position="inside">
<field name="custom_field"/>
</field>
</field>
</record>
</odoo>
6. To install your module, add it to the Odoo configuration file (`odoo.conf`) under the `addons_path` section. For example:
addons_path = /path/to/odoo/addons,/path/to/your/custom_module
7. Restart the Odoo server to apply your changes:
./odoo-bin -c odoo.conf
8. Go to the Odoo web interface, install and upgrade the `Custom Contacts Module` from the Apps menu. You should see the custom field added to the Contact form.
Step 12: Debugging Your Odoo Module
Debugging is an essential part of the development process. Visual Studio Code has great debugging capabilities for Python, and you can utilize them for Odoo development.
1. In your Visual Studio Code workspace, set breakpoints in your Python code.
2. Open the Debug panel in VS Code and create a new configuration for Python. Set the entry point to `./odoo-bin` and add the necessary arguments, e.g., `-c odoo.conf`.
3. Start the debugger, and you can now step through your Odoo code, inspect variables, and troubleshoot issues.
Step 13: Documenting Your Module
Documenting your module is crucial for maintaining and sharing your code. You can use Sphinx, a tool that makes it easy to generate documentation from your code comments.
1. Install Sphinx:
pip install sphinx
2. Create a `docs` directory in your module’s directory and run `sphinx-quickstart` to set up your documentation project.
3. Write documentation for your module in reStructuredText format within the `docs` directory.
4. Build the documentation by running:
sphinx-build -b html docs/ docs/_build
You now have a basic structure for documenting your module.
Conclusion
Configuring Odoo 15 in Visual Studio Code on Ubuntu 20.04 is not only about the initial setup but also about developing, debugging, and documenting your Odoo modules. By following these additional steps, you can create, debug, and document your modules efficiently, making your Odoo development workflow smoother and more organized. This robust development environment will help you work on customizations and extensions for Odoo with ease, allowing you to tailor it to your specific business needs.